How to build AI agents for beginners
This guide provides a detailed technical walkthrough for building ai agents for beginners with LangChain , complete with code examples, tool integration, and deployment strategies.The rapid evolution of large language models (LLMs) has enabled developers to create intelligent agents capable of reasoning, acting on external tools, and solving complex tasks. LangChain has emerged as the leading framework for building such agents, offering modular components for integrating LLMs with real-world data sources and APIs.
Foundational Concepts
What Are LangChain Agents?
LangChain agents are autonomous systems powered by LLMs that dynamically interact with tools (APIs, databases, calculators) to complete tasks. Unlike static chatbots, these agents:
- Reason about user queries using LLM capabilities
- Select tools based on contextual understanding
- Execute actions through predefined functions
- Iterate until reaching a satisfactory solution
Key components include the LLM core (e.g., GPT-4o, Claude 3.5) for decision-making, tools for task execution, an agent executor managing the action loop, and memory for context retention.
Development Setup
Environment Configuration
Create a Python environment (3.8+ required):
# Create Python environment (3.8+ required)
# Install core packages
API Key Management
Create a .env
file with the following content:
OPENAI_API_KEY='sk-your-key-here'
TAVILY_API_KEY='tvly-your-key-here' # For web searches
Building a Weather Information Agent
Tool Integration
Example code for integrating tools:
Agent Initialization
Initialize the agent with the LLM and tools:
Prompt Engineering
Define the system prompt and prompt template:
Execution and Testing
Running the Agent
Sample Output Analysis
The agent logs actions, such as querying for Tokyo’s current temperature and converting it from Celsius to Fahrenheit, before outputting the final answer.
Advanced Deployment
API Deployment with FastAPI
Adding Conversational Memory
Performance Optimization
Tool Selection Strategies
Error Handling
Evaluation Metrics
Metrics to consider include tool accuracy, response latency, error rate, and context retention across multi-turn conversations.
Future Development Paths
Multi-Agent Systems
RAG Integration
Conclusion
This guide demonstrates how to build a functional LangChain agent capable of integrating real-time data through API tools while maintaining conversational context. Key takeaways include:
- Modular architecture enables flexible tool integration
- Prompt engineering significantly impacts agent performance
- Memory management is crucial for complex workflows
- Error handling ensures production reliability
Future enhancements may incorporate multimodal inputs, real-time data streams, and reinforcement learning for self-improvement. Developers should continually evaluate new LangChain features and LLM capabilities to stay at the cutting edge of AI agent development.
Final Complete Implementation
About the Author
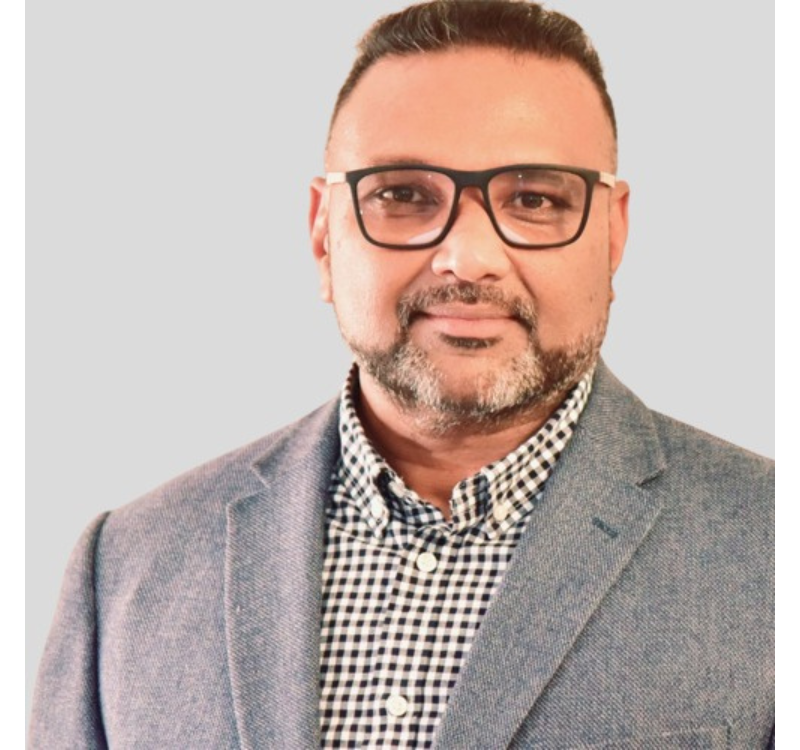
Rejith Krishnan
Rejith Krishnan is the Founder and CEO of lowtouch.ai, a platform dedicated to empowering enterprises with private, no-code AI agents. With expertise in Site Reliability Engineering (SRE), Kubernetes, and AI systems architecture, he is passionate about simplifying the adoption of AI-driven automation to transform business operations.
Rejith specializes in deploying Large Language Models (LLMs) and building intelligent agents that automate workflows, enhance customer experiences, and optimize IT processes, all while ensuring data privacy and security. His mission is to help businesses unlock the full potential of enterprise AI with seamless, scalable, and secure solutions that fit their unique needs.